If you are a frontend developer, you might have heard of Redux. If you are not a frontend developer, or you have not heard of Redux, this article will still enlighten you on a cool piece of tech that your fellow frontend engineers use.
New React developers learn all the React fundamentals, but are often scared of diving into Redux. The reason for this is mostly the confusing terminologies that Redux brings with it along with a steeper learning curve than traditional JavaScript libraries and frameworks. This article aims to clear the air by explaining Redux jargon with a real world example.
What is Redux?
First things first, Redux is not bound with React. It can be used with Vanilla JavaScript, Vue, Angular, and other fancy JS Frameworks. React-Redux on the other hand, is a React specific package that makes working with Redux easier in React applications. Therefore, due to React’s popularity and my personal experience with it, in this article we will be talking about Redux from React’s point of view. However, the overall concepts and terminologies remain similar.
So, what is Redux?
Redux is a state management library for JavaScript applications.
Okay? What does that even mean?
Every web application you see has an inherent state, or a set of properties that differ between users and might be dependent on user interactions.
When you visit Amazon, the state of the app holds details of the user which is logged in, the items in your cart, if a modal is open or not, etc. This information is used in and can be updated from various places throughout the app. For example, you can add items to your cart from the products page and delete them from the cart page.
Redux is responsible for storing these pieces of information so that they can be accessed by respective components of the app whenever needed.
Why Redux?
If you have used React, you know that each component has its own state. So why use Redux when we can directly manipulate the state of the component?
Let’s say you have a React app with multiple components nested within each other. The component D needs to display the name of the user, so it has a state variable with value “Apoorva”
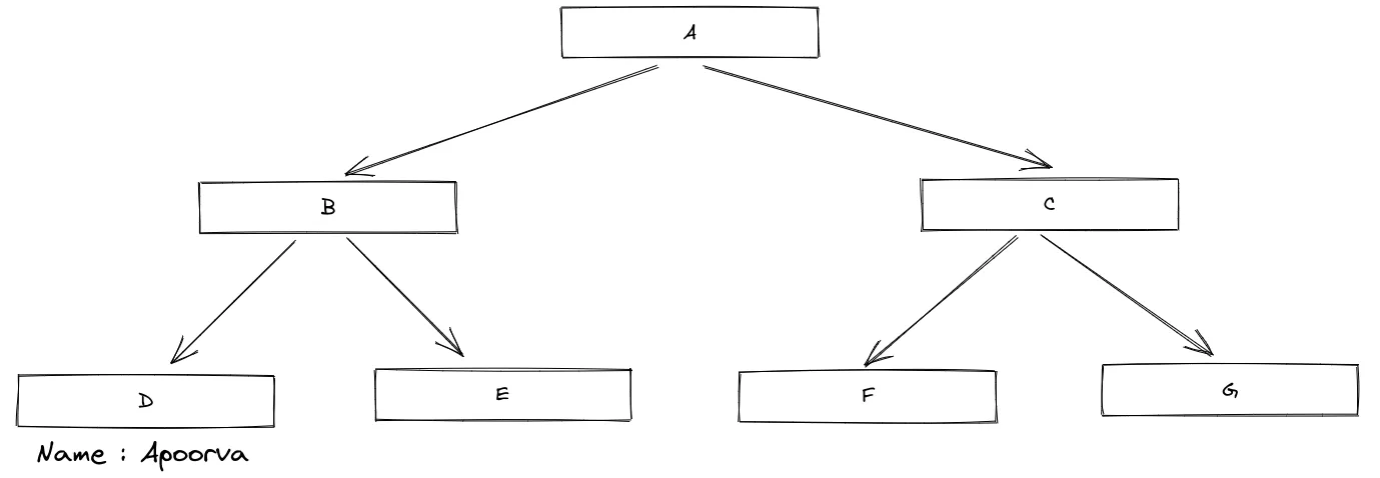
After we develop some more features, we find out that component E also requires to display the name. By using React logic, we lift up the variable and store it in parent component B that can then pass it to its children (D,E).
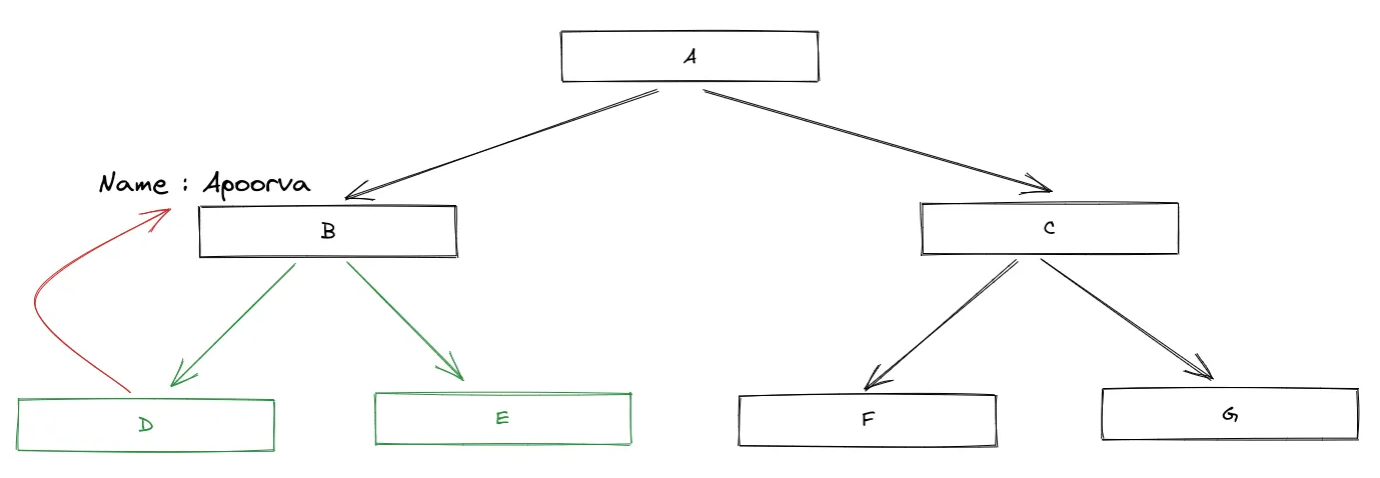
After some more development, we find out that component G also requires the name of the user. Going again by our logic, we lift up the variable and store it in the least common ancestor of the children components (A in our case).
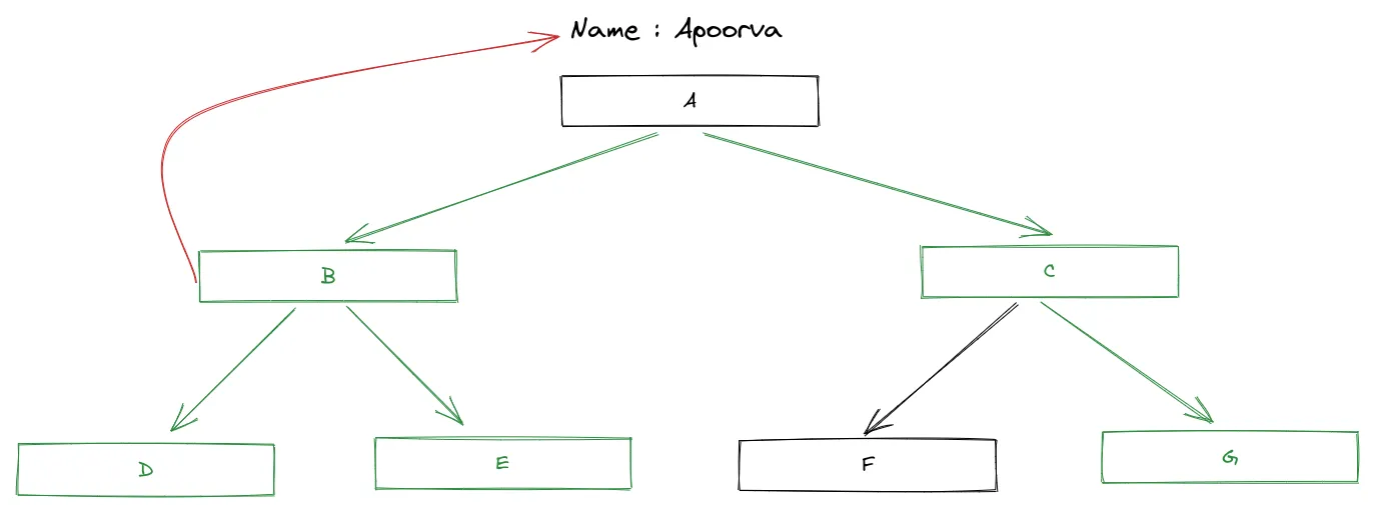
We can see the obvious problems with this approach.
1. Imagine doing this with multiple properties at different levels of nesting. It becomes a very confusing and tedious task for the developer, as well as decreases code readability.
2. Components B and C, which have no use of the variable, must also store its value only because their children need it.
Thus, Redux comes to our rescue by providing us an external reference to the application state, which can be queried by any component that requires a state variable.
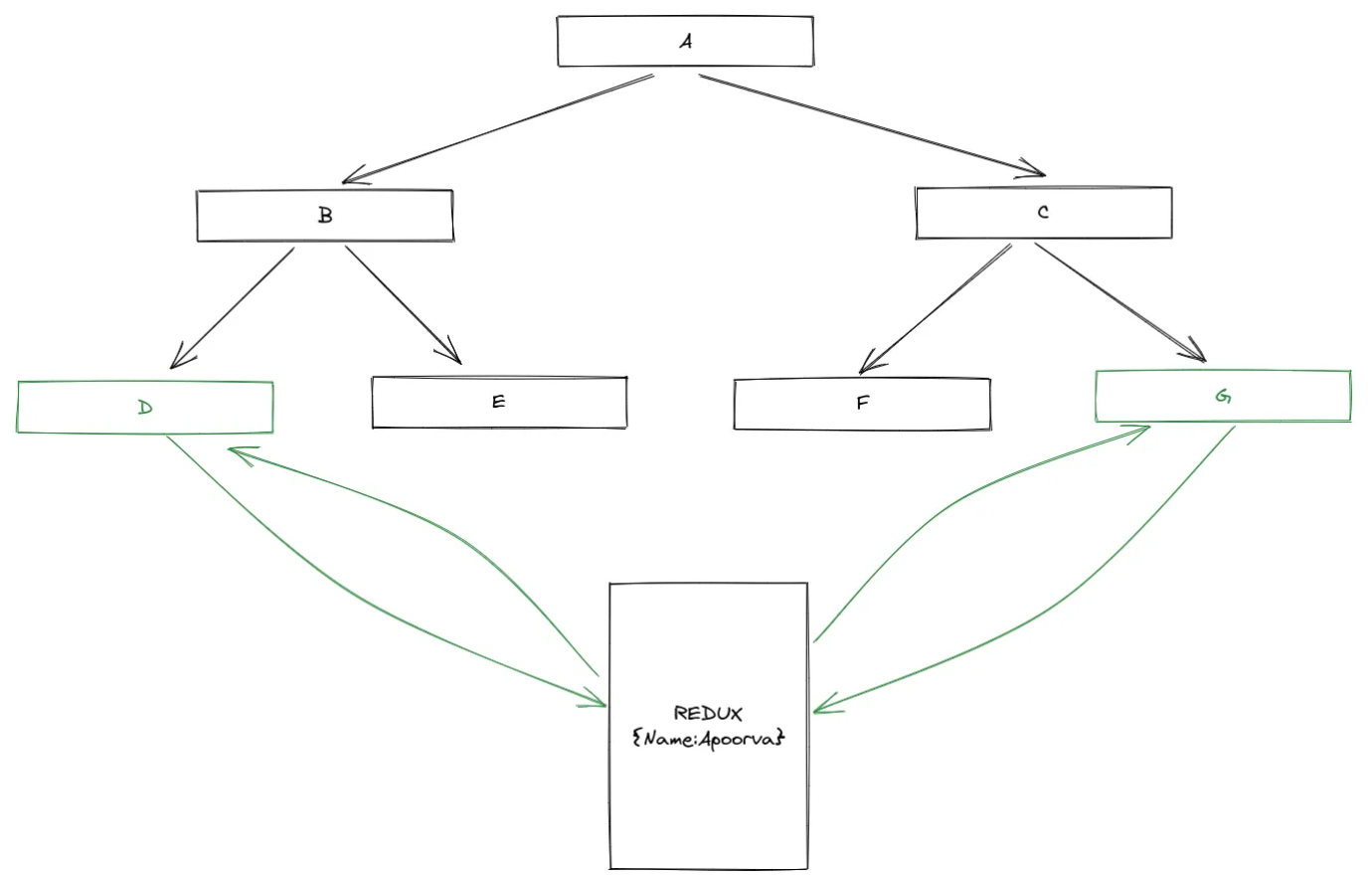
Redux might seem like a database since it has similar functionality, but Redux is solely responsible for holding data in memory to be displayed on the app. Querying the database for the same information is a redundant and time consuming task.
Additionally, some of you may be wondering if the UseContext and UseReducer hooks in React can do the same job. This is correct. Redux, however, was developed before these hooks and has thus been in use for longer. Apart from being framework agnostic, it is still the recommended way to handle app state on larger scale.
Redux Terminologies
Redux has 3 key terms:
Store, Actions, and Reducers.
These terms follow the 3 principles of Redux, if none of them make sense, trust me and keep reading. :
1. State of the application is stored in an object tree with a single store.
2. The only way to change the store is to emit an action, which is an object describing what needs to happen.
3. To specify how the state tree is transformed, we need to write reducers that take initial state, and an action as input and return the new state.
Let’s say you received your first salary and want to buy a new laptop.
You go to the laptop shop and tell the shopkeeper you want to purchase a laptop.
The shop here is our JavaScript application and the shelf is the state of the application (Redux Store). Let’s say the shelf is used to keep a track of how many laptops are in the shop along with their details. This information is the state of our application.
You telling the shopkeeper you need to buy a laptop is the Redux Action. It specifies how the Redux store must be changed. (Reduce the number of laptops by 1)
The shopkeeper checks if that particular model is on the shelf or not.
The shopkeeper is the Redux Reducer.
ONLY the shopkeeper can access the shelf and change its content. That is, ONLY the Reducer can manipulate the Redux store. You cannot directly change the contents of the Redux store.
Sure, you can jump the counter and take the laptop yourself while leaving some money behind, but that is not advisable and not the conventional practice, unless you want criminal charges XD
Let’s say, after some days, you realise the laptop needs more RAM so you come back to the shop to upgrade it. While you are there, you figure out that you need a phone as well, so you go to the other shopkeeper on the phone counter and buy the phone you need.
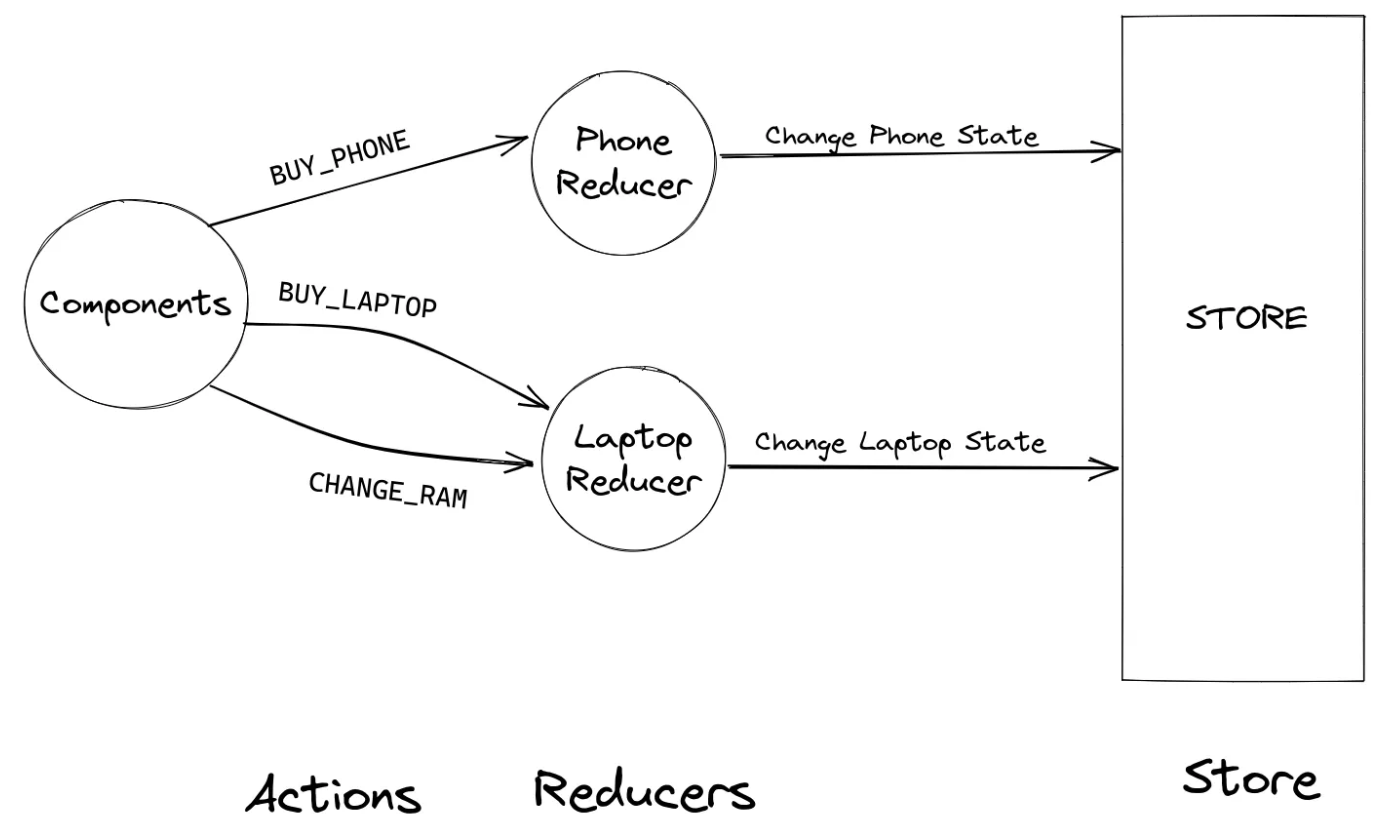
The shop could have had one shopkeeper to handle both laptops and phones, but it would get difficult to manage once the shop starts selling more items. Therefore, multiple reducers can be used to change the state of the same Redux store, because using a single reducer is difficult to scale. The function signature for reducers is given below:
Note that the functions inside the cases should ideally be implemented within the case itself, instead of being called. They are abstracted here for the sake of brevity.
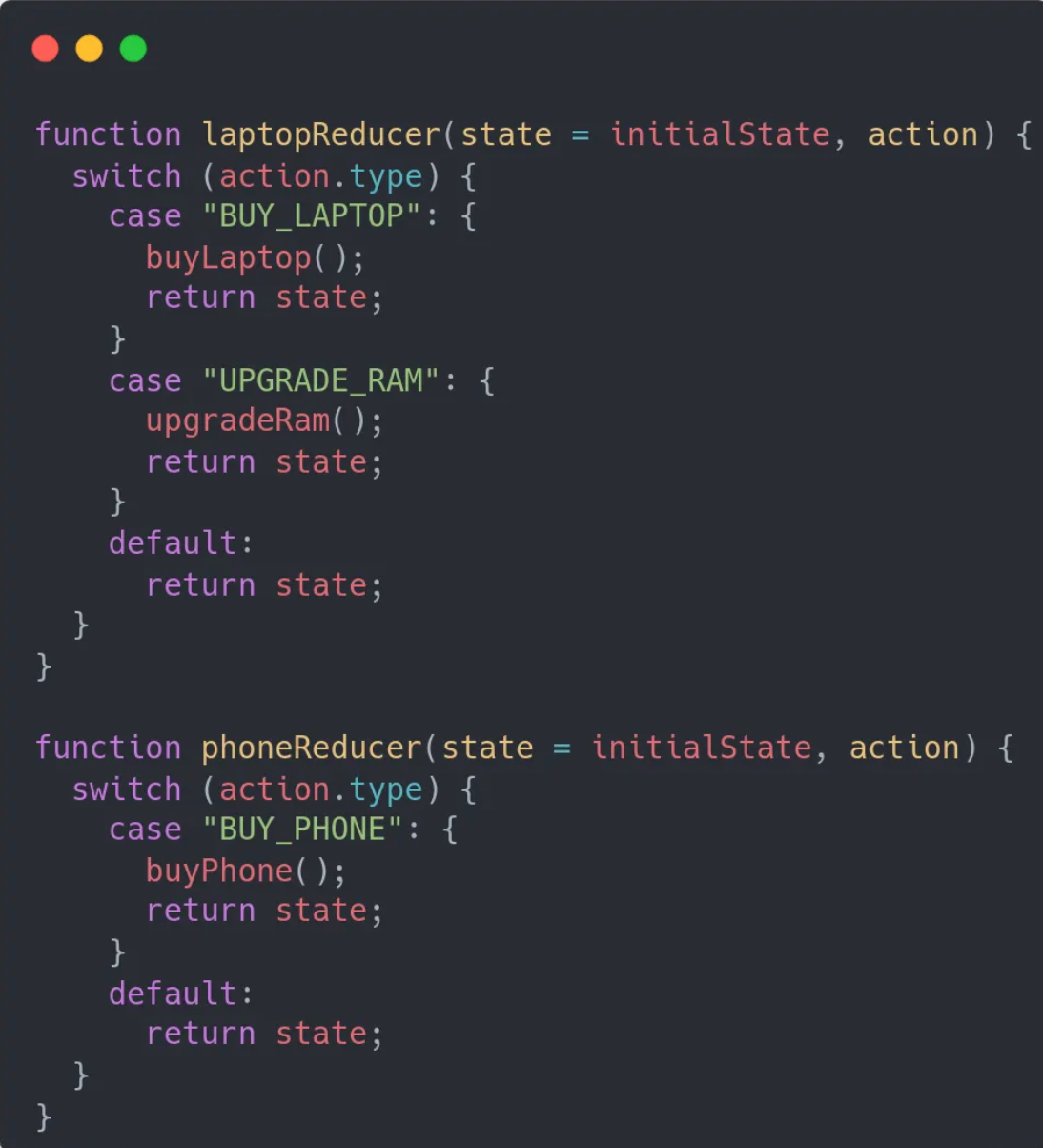
. . .
So if you are a frontend developer, I hope you see now that even though the initial learning curve is steep, once you get over it, Redux will make your development experience much smoother, organised, and efficient.
I would highly recommend to practise by implementing Redux in a simple application like the infamous “ToDo List”, because no matter how many articles you read, if you don’t mess around yourself, you won’t find anything out.